Zepcode alternatives and similar libraries
Based on the "Editors" category.
Alternatively, view Zepcode alternatives based on common mentions on social networks and blogs.
-
medium-editor
Medium.com WYSIWYG editor clone. Uses contenteditable API to implement a rich text solution. -
TinyMCE
The world's #1 JavaScript library for rich text editing. Available for React, Vue and Angular -
CKEditor 5
Powerful rich text editor framework with a modular architecture, modern integrations, and features like collaborative editing. -
SimpleMDE
A simple, beautiful, and embeddable JavaScript Markdown editor. Delightful editing for beginners and experts alike. Features built-in autosaving and spell checking. -
wysihtml5
DISCONTINUED. Open source rich text editor based on HTML5 and the progressive-enhancement approach. Uses a sophisticated security concept and aims to generate fully valid HTML5 markup by preventing unmaintainable tag soups and inline styles. -
EpicEditor
EpicEditor is an embeddable JavaScript Markdown editor with split fullscreen editing, live previewing, automatic draft saving, offline support, and more. For developers, it offers a robust API, can be easily themed, and allows you to swap out the bundled Markdown parser with anything you throw at it. -
Materio Free Vuetify VueJS Admin Template
Production Ready, Carefully Crafted, Extensive Vuetifty Free Admin Template 🤩 -
Bangle.dev
Collection of higher level rich text editing tools. It powers the local only note taking app https://bangle.io -
Everright-formEditor
:guide_dog: Powerful lowcode|vue form editor,generator,designer,builder library. It provides an easy way to create custom forms. The project is extensible, easy to use and configure, and provides many commonly used form components and functions(vue可视化低代码表单设计器、表单编辑器、element-plus vant表单设计) -
React Chat UI
Build your own chat UI with React Chat UI components in a few minutes. React Chat UI Kit from minchat.io is an open source UI toolkit for developing web chat applications. -
data-structure-typed
Javascript Data Structure & TypeScript Data Structure. Heap, Binary Tree, Red Black Tree, Linked List, Deque, Trie, HashMap, Directed Graph, Undirected Graph, Binary Search Tree, AVL Tree, Priority Queue, Graph, Queue, Tree Multiset, Singly Linked List, Doubly Linked List, Max Heap, Max Priority Queue, Min Heap, Min Priority Queue, Stack.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
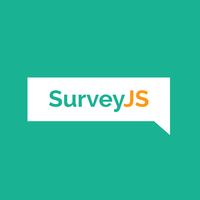
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Zepcode or a related project?
README
Zeplin extension that generates Swift snippets from colors, fonts and layers.
Features
- 🖍 Color pallette for iOS
Example
import UIKit
extension UIColor {
static let electricBlue = UIColor(red: 0/255, green: 86/255, blue: 255/255, alpha: 1)
}
Example with custom initializer
import UIKit
extension UIColor {
convenience init(r red: Int, g green: Int, b blue: Int, a: CGFloat = 1) { // swiftlint:disable:this identifier_name
self.init(red: CGFloat(red) / 255,
green: CGFloat(green) / 255,
blue: CGFloat(blue) / 255,
alpha: a)
}
static let electricBlue = UIColor(r: 0, g: 86, b: 255)
}
Example with color literals
import UIKit
extension UIColor {
static let electricBlue = #colorLiteral(red: 0, green: 0.337254902, blue: 1, alpha: 1)
}
- ✏️ Fonts for iOS
Example
import UIKit
extension UIFont {
static func BloggerSansBold(ofSize: CGFloat) -> UIFont {
return UIFont(name: "BloggerSans-Bold", size: size)!
}
}
- 🚧 Snippets for borders and corner radius
Example
view.layer.borderWidth = 4
view.layer.borderColor = UIColor.white.cgColor
view.layer.cornerRadius = 40
- 🌚 Snippets for shadows
Example
view.layer.shadowColor = UIColor(r: 0, g: 0, b: 0, a: 0.5).cgColor
view.layer.shadowOpacity = 1
view.layer.shadowOffset = CGSize(width: 0, height: 2)
view.layer.shadowRadius = 4 / 2
let rect = view.bounds.insetBy(dx: -2, dy: -2)
view.layer.shadowPath = UIBezierPath(rect: rect).cgPath
- 🎨 Gradients (Work in progress)
Linear gradient example
Check out [LinearGradientPlayground](.github/LinearGradientPlayground.playground) and read explanation of the implementation here.
Radial gradient example
final class RadialGradientView: UIView {
private var radius: CGFloat {
return min(bounds.width / 2, bounds.height / 2)
}
private let colors = [UIColor.red.cgColor, UIColor.neonGreen.cgColor]
var options: CGGradientDrawingOptions = CGGradientDrawingOptions(rawValue: 0)
// MARK: - Lifecycle
override init(frame: CGRect) {
super.init(frame: frame)
clipsToBounds = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func layoutSubviews() {
super.layoutSubviews()
layer.cornerRadius = radius
}
override func draw(_ rect: CGRect) {
let context = UIGraphicsGetCurrentContext()
let colorSpace = CGColorSpaceCreateDeviceRGB()
let colorsCount = colors.count
var locations = (0..<colorsCount).map { i in
return CGFloat(i) / CGFloat(colorsCount)
}
guard let gradient = CGGradient(colorsSpace: colorSpace, colors: colors as CFArray, locations: locations) else {
return
}
context?.drawRadialGradient(gradient,
startCenter: center,
startRadius: 0,
endCenter: center,
endRadius: radius,
options: options)
}
}
Options
Use color names
Use color names from Color Palette or default UIColor(red:green:blue:alpha:)
initializers.
Initializer style
- Default — Use the default
UIColor(red:green:blue:alpha:)
initializer. - Custom — Use
UIColor(r:g:b:a:)
initializer. - Literal — Use color literals
#colorLiteral(red:green:blue:alpha:)
that will appear in Xcode as a colored rect that presents a color picker.
Use layer extension for shadows
Use a function below for shadow parameters. Don't forget to add [this extension](.github/CALayer+Shadow.swift) to your project.
import UIKit
extension CALayer {
func makeShadow(color: UIColor,
x: CGFloat = 0,
y: CGFloat = 0,
blur: CGFloat = 0,
spread: CGFloat = 0) {
shadowColor = color.cgColor
shadowOpacity = 1
shadowOffset = CGSize(width: x, height: y)
shadowRadius = blur / 2
if spread == 0 {
shadowPath = nil
}
else {
let rect = bounds.insetBy(dx: -spread, dy: -spread)
shadowPath = UIBezierPath(rect: rect).cgPath
}
}
}
How to Install
Zepcode is available on [Zeplin Extensions](extensions.zeplin.io).
How to make a changes
First, you need last stable Node.js ^8.9.4
. Follow this guide if you don't have any.
Next, install project dependencies:
npm i
To start developing, to make a build or to execute some functions from extension follow this guide.
To learn more about zem, see documentation.
If you like to take full control of your build process you can try zero boilerplate.
Authors
Artem Novichkov, [email protected]
Baybara Pavel, [email protected]
License
Zepcode is available under the MIT license. See the LICENSE file for more info.
*Note that all licence references and agreements mentioned in the Zepcode README section above
are relevant to that project's source code only.