Description
The default JavaScript tracking snippet for Google Analytics runs when a web page is first loaded and sends a pageview hit to Google Analytics. If you want to know about more than just pageviews (e.g. events, social interactions), you have to write code to capture that information yourself.
Since most website owners care about a lot of the same types of user interactions, web developers end up writing the same code over and over again for every new site they build.
Autotrack was created to solve this problem. It provides default tracking for the interactions most people care about, and it provides several convenience features (e.g. declarative event tracking) to make it easier than ever to understand how people are using your site.
Autotrack alternatives and similar libraries
Based on the "Misc" category.
Alternatively, view Autotrack alternatives based on common mentions on social networks and blogs.
-
list.js
The perfect library for adding search, sort, filters and flexibility to tables, lists and various HTML elements. Built to be invisible and work on existing HTML. -
InversifyJS
A powerful and lightweight inversion of control container for JavaScript & Node.js apps powered by TypeScript. -
mixitup
A high-performance, dependency-free library for animated filtering, sorting, insertion, removal and more -
surveyjs
Free Open-Source JavaScript form builder library with integration for React, Angular, Vue, jQuery, and Knockout that lets you load and run multiple web forms, or build your own self-hosted form management system, retaining all sensitive data on your servers. You have total freedom of choice as to the backend, because any server + database combination is fully compatible.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
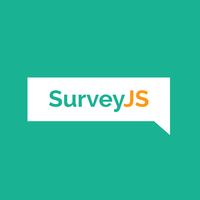
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Autotrack or a related project?
README
This library is no longer actively maintained
Autotrack 
Overview
The default JavaScript tracking snippet for Google Analytics runs when a web page is first loaded and sends a pageview hit to Google Analytics. If you want to know about more than just pageviews (e.g. where the user clicked, how far they scroll, did they see certain elements, etc.), you have to write code to capture that information yourself.
Since most website owners care about a lot of the same types of user interactions, web developers end up writing the same code over and over again for every new site they build.
Autotrack was created to solve this problem. It provides default tracking for the interactions most people care about, and it provides several convenience features (e.g. declarative event tracking) to make it easier than ever to understand how people are interacting with your site.
Plugins
The autotrack.js
file in this repository is small (8K gzipped) and comes with all plugins included. You can use it as is, or you can create a custom build that only includes the plugins you want to make it even smaller.
The following table briefly explains what each plugin does; you can click on the plugin name to see the full documentation and usage instructions:
Plugin Description cleanUrlTracker Ensures consistency in the URL paths that get reported to Google Analytics; avoiding the problem where separate rows in your pages reports actually point to the same page. eventTracker Enables declarative event tracking, via HTML attributes in the markup. impressionTracker Allows you to track when elements are visible within the viewport. maxScrollTracker Automatically tracks how far down the page a user scrolls. mediaQueryTracker Enables tracking media query matching and media query changes. outboundFormTracker Automatically tracks form submits to external domains. outboundLinkTracker Automatically tracks link clicks to external domains. pageVisibilityTracker Automatically tracks how long pages are in the visible state (as opposed to in a background tab) socialWidgetTracker Automatically tracks user interactions with the official Facebook and Twitter widgets. urlChangeTracker Automatically tracks URL changes for single page applications.
Disclaimer: autotrack is maintained by members of the Google Analytics developer platform team and is primarily intended for a developer audience. It is not an official Google Analytics product and does not qualify for Google Analytics 360 support. Developers who choose to use this library are responsible for ensuring that their implementation meets the requirements of the Google Analytics Terms of Service and the legal obligations of their respective country.
Installation and usage
To add autotrack to your site, you have to do two things:
- Load the
autotrack.js
script file included in this repo (or a custom build) on your page. - Update your tracking snippet to require the various autotrack plugins you want to use on the tracker.
If your site is currently using the default JavaScript tracking snippet, you can modify it to something like this:
<script>
window.ga=window.ga||function(){(ga.q=ga.q||[]).push(arguments)};ga.l=+new Date;
ga('create', 'UA-XXXXX-Y', 'auto');
// Replace the following lines with the plugins you want to use.
ga('require', 'eventTracker');
ga('require', 'outboundLinkTracker');
ga('require', 'urlChangeTracker');
// ...
ga('send', 'pageview');
</script>
<script async src="https://www.google-analytics.com/analytics.js"></script>
<script async src="path/to/autotrack.js"></script>
Of course, you'll have to make the following modifications to the above code to customize autotrack to your needs:
- Replace
UA-XXXXX-Y
with your tracking ID - Replace the sample list of plugin
require
statements with the plugins you want to use. - Replace
path/to/autotrack.js
with the actual location of theautotrack.js
file hosted on your server.
Note: the analytics.js plugin system is designed to support asynchronously loaded scripts, so it doesn't matter if autotrack.js
is loaded before or after analytics.js
. It also doesn't matter if the autotrack.js
library is loaded individually or bundled with the rest of your JavaScript code.
Loading autotrack via npm
If you use npm and a module loader that understands ES2015 imports (e.g. Webpack, Rollup, or SystemJS), you can include autotrack in your build by importing it as you would any other npm module:
npm install autotrack
// In your JavaScript code
import 'autotrack';
Note: autotrack's source is published as ES2015, and you will need to make sure you're not excluding it from compilation. See #137 for more details.
The above import
statement will include all autotrack plugins in your generated source file. If you only want to include a specific set of plugins, you can import them individually:
// In your JavaScript code
import 'autotrack/lib/plugins/event-tracker';
import 'autotrack/lib/plugins/outbound-link-tracker';
import 'autotrack/lib/plugins/url-change-tracker';
The above examples show how to include the autotrack plugin source in your site's main JavaScript bundle, which accomplishes the first step of the two-step installation process. However, you still have to update your tracking snippet and require the plugins you want to use on the tracker.
// Import just the plugins you want to use.
import 'autotrack/lib/plugins/event-tracker';
import 'autotrack/lib/plugins/outbound-link-tracker';
import 'autotrack/lib/plugins/url-change-tracker';
ga('create', 'UA-XXXXX-Y', 'auto');
// Only require the plugins you've imported above.
ga('require', 'eventTracker');
ga('require', 'outboundLinkTracker');
ga('require', 'urlChangeTracker');
ga('send', 'pageview');
Code splitting
Note that it's generally not a good idea to include any analytics as part of your site's main JavaScript bundle since analytics are not usually critical application functionality.
If you're using a bundler that supports code splitting (via something like System.import()
), it's best to load autotrack plugins lazily and delay their initialization until after your site's critical functionality has loaded:
window.addEventListener('load', () => {
const autotrackPlugins = [
'autotrack/lib/plugins/event-tracker',
'autotrack/lib/plugins/outbound-link-tracker',
'autotrack/lib/plugins/url-change-tracker',
// List additional plugins as needed.
];
Promise.all(autotrackPlugins.map((x) => System.import(x))).then(() => {
ga('create', 'UA-XXXXX-Y', 'auto');
ga('require', 'eventTracker', {...});
ga('require', 'outboundLinkTracker', {...});
ga('require', 'urlChangeTracker', {...});
// Require additional plugins imported above.
ga('send', 'pageview');
});
})
If you're not sure how do use code splitting with your build setup, see the custom builds section to learn how to manually generate a custom version of autotrack with just the plugins you need.
Passing configuration options
All autotrack plugins accept a configuration object as the third parameter to the require
command.
Some of the plugins (e.g. outboundLinkTracker
, socialWidgetTracker
, urlChangeTracker
) have a default behavior that works for most people without specifying any configuration options. Other plugins (e.g. cleanUrlTracker
, impressionTracker
, mediaQueryTracker
) require certain configuration options to be set in order to work.
See the individual plugin documentation to reference what options each plugin accepts (and what the default value is, if any).
Advanced configuration
Custom builds
Autotrack comes with its own build system, so you can create autotrack bundles containing just the plugins you need. Once you've installed autotrack via npm, you can create custom builds by running the autotrack
command.
For example, the following command generates an autotrack.js
bundle and source map for just the eventTracker
, outboundLinkTracker
, and urlChangeTracker
plugins:
autotrack -o path/to/autotrack.custom.js -p eventTracker,outboundLinkTracker,urlChangeTracker
Once this file is generated, you can include it in your HTML templates where you load analytics.js
. Note the use of the async
attribute on both script tags. This prevents analytics.js
and autotrack.custom.js
from interfering with the loading of the rest of your site.
<script async src="https://www.google-analytics.com/analytics.js"></script>
<script async src="path/to/autotrack.custom.js"></script>
Using autotrack with multiple trackers
All autotrack plugins support multiple trackers and work by specifying the tracker name in the require
command. The following example creates two trackers and requires various autotrack plugins on each.
// Creates two trackers, one named `tracker1` and one named `tracker2`.
ga('create', 'UA-XXXXX-Y', 'auto', 'tracker1');
ga('create', 'UA-XXXXX-Z', 'auto', 'tracker2');
// Requires plugins on tracker1.
ga('tracker1.require', 'eventTracker');
ga('tracker1.require', 'socialWidgetTracker');
// Requires plugins on tracker2.
ga('tracker2.require', 'eventTracker');
ga('tracker2.require', 'outboundLinkTracker');
ga('tracker2.require', 'pageVisibilityTracker');
// Sends the initial pageview for each tracker.
ga('tracker1.send', 'pageview');
ga('tracker2.send', 'pageview');
Browser Support
Autotrack will safely run in any browser without errors, as feature detection is always used with any potentially unsupported code. However, autotrack will only track features supported in the browser running it. For example, a user running Internet Explorer 8 will not be able to track media query usage, as media queries themselves aren't supported in Internet Explorer 8.
All autotrack plugins are tested in the following browsers:
✔ ✔ 6+ ✔ 9+ ✔
Translations
The following translations have been graciously provided by the community. Please note that these translations are unofficial and may be inaccurate or out of date:
If you discover issues with a particular translation, please file them with the appropriate repository. To submit your own translation, follow these steps:
- Fork this repository.
- Update the settings of your fork to allow issues.
- Remove all non-documentation files.
- Update the documentation files with your translated versions.
- Submit a pull request to this repository that adds a link to your fork to the above list.